2020~2022工作笔记
Pycharm常用快捷键
- ctrl + e 查看最近编辑文件
- ctrl + shift + r 根据文件名查找文件
- ctrl + h 全局搜索
- ctrl + alt + o 优化导入包排序
- ctrl + shift + o 开启翻译框
快捷键设置:
放大、缩小设置:File ->setting-> Keymap->搜索框中输入:Increase Font Size(双击选中)->弹出对话框时选择Add Mouse Shortcut(单击)
json、pickle、shelve 各自数据序列化方式的异同和用法
json适用于不同类型的语言,支持的数据类型:int\str\list\tuple\dict
主要是操作字符串dumps、loads
操作 字符串dump、load
操作 文件 (pickle一样的情况)
1 | # dump入文件 |
pickle 专为python设计,支持python所有的数据类型,主要是操作字节流
1 | pickle.dumps("cxs") |
用 python 写入 csv 后的中文乱码问题
__init__.py 文件的作用
- 让一个普通文件夹变成一个python模块
- 在import一个模块时,__init__文件最先被执行
json.dumps 参数
indent 保持缩进
ensure_ascii 防止汉字被转换为 unicode (多用于写入文件时)
1
2
3
4
5
6
7
8
9
10
11
12
13d = {"name": "陈晓生", "age": 24}
print(json.dumps(d, indent=4, ensure_ascii=False))
{
"name": "陈晓生",
"age": 24
}
print(json.dumps(d, indent=4))
{
"name": "\u9648\u6653\u751f",
"age": 24
}separators 自定义分隔符
1
separators=(",", ":")
ciphey 基于深度学习自动解密密文
docker容器和镜像
镜像是应用
的运行环境
容器是运行环境+应用
即容器是镜像的应用实例
常用命令
docker inspect
查看容器信息docker run
部署容器docker start / restart / stop
容器文件拷贝到宿主机
docker cp 容器id:容器内文件路径 目标路径
1
docker cp 4783c8deb107:/root/kf/DY/select_shop.png /tmp/cxs.png
python项目导出requirements.txt
参考:https://learnku.com/articles/47470
1 | pip install pipreqs |
添加文件头,指定库地址
1 | --index-url=https://mirrors.aliyun.com/pypi/simple/ --trusted-host mirrors.aliyun.com |
如何安装?
pycharm - Terminal - pip3 install -r requirements.txt
Payload
和Form Data
- Payload Content-Type:application/json
1 | from scrapy.http import JsonRequest |
- FormData Content-Type:applicatin/x-www-form-urlencode
1 | yield scrapy.FormRequest(formdata=data) |
JWT原理(JSON WEB TOKEN)
参考:https://www.cnblogs.com/fengzheng/p/13527425.html
常用于单点登录场景,数据由头部、载荷与签名这三部分组成,中间以 .
分隔,
- 头部 使用base64 encode,指明令牌类型和加密算法
- 载荷 使用base64 encode,用来存储服务器需要的数据
- 签名 使用
HMACSHA256算法
,secret key 存储在服务端
代码示例
1 | login_url = "https://login3.scrape.center/api/login" |
WebKitFormBoundary文件上传
1 | from requests_toolbelt import MultipartEncoder |
实现效果:
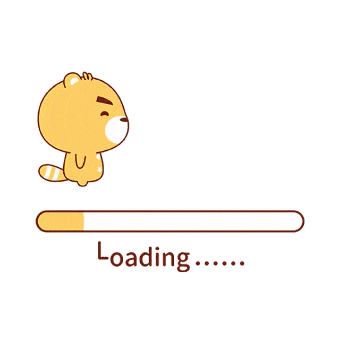
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 Chen's Blog!
评论